C++控制台應用程序之貪吃蛇(改進版),貪吃蛇改進版
1 #include<iostream>
2 #include<stdio.h>
3 #include<stdlib.h>
4 #include<time.h>
5 #include<conio.h>
6 #include<windows.h>
7 using namespace std;
8
9 typedef struct{ int x, y; }Point;
10
11 char map[22][22]; //定義一個22*22的地圖(含邊界)
12 Point snake[400], food, Next; //定義蛇、食物、下一步蛇頭的位置
13 int head, tail; //用於儲存蛇頭和蛇尾的下標
14 int grade, length, autotime; //游戲等級、蛇長、自動前進所需時間
15 char direction; //前進方向
16
17 //定位光標
18 void gotoxy(int x, int y)
19 {
20 HANDLE hConsoleOutput;
21 COORD dwCursorPosition;
22 dwCursorPosition.X = x;
23 dwCursorPosition.Y = y;
24 hConsoleOutput = GetStdHandle(STD_OUTPUT_HANDLE);
25 SetConsoleCursorPosition(hConsoleOutput, dwCursorPosition);
26 }
27
28 //用inline定義內聯函數節省程序運行時的調用開銷
29 //刷新地圖
30 inline void Update(char map[][22], int grade, int length, int autotime)
31 {
32 //system("cls"); //清屏
33 gotoxy(0, 0);
34 int i, j;
35 printf("\n");
36 for (i = 0; i < 22; i++)
37 {
38 printf("\t");
39 for (j = 0; j < 22; j++)
40 printf("%c ", map[i][j]);
41 if (i == 0)
42 printf("\t等級為:%d", grade);
43 if (i == 2)
44 printf("\t長度為:%d", length);
45 if (i == 6)
46 printf("\t自動前進時間");
47 if (i == 8)
48 printf("\t間隔為:%d ms", autotime);
49 printf("\n");
50 }
51 }
52
53 //歡迎界面
54 inline void hello()
55 {
56 puts("\n\n\n\t\t\t貪吃蛇游戲即將開始!"); //准備開始
57 double start;
58 for (int i = 3; i >= 0; i--)
59 {
60 start = (double)clock() / CLOCKS_PER_SEC; //得到程序目前為止運行的時間
61 while ((double)clock() / CLOCKS_PER_SEC - start <= 1); //經過1秒之後
62 if (i > 0)
63 {
64 system("cls"); //清屏
65 printf("\n\n\n\t\t\t進入倒計時:%d\n", i); //倒計時
66 }
67 else
68 Update(map, grade, length, autotime); //刷新地圖
69 }
70 }
71
72 //隨機生成食物位置
73 inline void f()
74 {
75 srand(int(time(0))); //調用種子函數
76 do{
77 food.x = rand() % 20 + 1;
78 food.y = rand() % 20 + 1;
79 } while (map[food.x][food.y] != ' ');
80 map[food.x][food.y] = '!'; //食物為“!”
81 }
82
83 //初始化
84 inline void init()
85 {
86 int i, j;
87 for (i = 1; i <= 20; i++)
88 for (j = 1; j <= 20; j++)
89 map[i][j] = ' ';
90 for (i = 0; i <= 21; i++)
91 map[0][i] = map[21][i] = map[i][0] = map[i][21] = '*'; //邊界
92 map[1][1] = map[1][2] = 'O'; //蛇身(含蛇尾)
93 map[1][3] = '@'; //蛇頭
94 head = 2; tail = 0; //開始時頭和尾的下標
95 snake[head].x = 1; snake[head].y = 3; //開始時蛇頭在地圖上的下標
96 snake[tail].x = 1; snake[tail].y = 1; //開始時蛇尾在地圖上的下標
97 snake[1].x = 1; snake[1].y = 2; //開始時蛇身在地圖上的下標
98 f(); //隨機生成食物位置
99 grade = 1; length = 3; autotime = 500; //開始的等級、長度、自動前進時間
100 direction = 77; //初始的運動方向向右
101 }
102
103 //預前進
104 inline int GO()
105 {
106 bool timeover = true;
107 double start = (double)clock() / CLOCKS_PER_SEC; //得到程序目前為止運行的時間
108
109 L:
110 //自動經過1秒或者等待1秒內的鍵盤輸入
111 while ((timeover = ((double)clock() / CLOCKS_PER_SEC - start <= autotime / 1000.0)) && !_kbhit());
112 //鍵盤輸入
113 if (timeover)
114 {
115 //_getch();
116 char d = _getch(); //獲取方向
117 if (d != 72 && d != 80 && d != 75 && d != 77 || direction == 72 && d == 80 || direction == 80 && d == 72 || direction == 75 && d == 77 || direction == 77 && d == 75)
118 goto L;
119 else
120 direction = d;
121 }
122 switch (direction)
123 {
124 case 72:
125 Next.x = snake[head].x - 1; Next.y = snake[head].y; //向上
126 break;
127 case 80:
128 Next.x = snake[head].x + 1; Next.y = snake[head].y; //向下
129 break;
130 case 75:
131 Next.x = snake[head].x; Next.y = snake[head].y - 1; //向左
132 break;
133 case 77:
134 Next.x = snake[head].x; Next.y = snake[head].y + 1; //向右
135 break;
136 default:
137 puts("\tGame over!"); //按下非方向鍵游戲失敗
138 return 0;
139 }
140 if (Next.x == 0 || Next.x == 21 || Next.y == 0 || Next.y == 21) //撞到邊界
141 {
142 puts("\tGame over!");
143 return 0;
144 }
145 if (map[Next.x][Next.y] != ' '&&!(Next.x == food.x&&Next.y == food.y)) //吃到自己
146 {
147 puts("\tGame over!");
148 return 0;
149 }
150 if (length == 400) //最長蛇長
151 {
152 puts("\tGood game!");
153 return 0;
154 }
155 return 1;
156 }
157
158 //吃到食物
159 inline void EAT()
160 {
161 length++; //長度增加1
162 int _grade = length / 10 + 1; //計算等級
163 if (_grade != grade)
164 {
165 grade = _grade;
166 if (autotime >= 100)
167 autotime = 550 - grade * 50; //增加一級自動時間減短50毫秒
168 }
169 map[Next.x][Next.y] = '@'; //蛇頭位置變化
170 map[snake[head].x][snake[head].y] = 'O'; //原蛇頭位置變化為蛇身
171 head = (head + 1) % 400; //蛇頭下標加1
172 snake[head].x = Next.x; snake[head].y = Next.y; //蛇頭下標變化
173 f(); //隨機生成食物位置
174 Update(map, grade, length, autotime); //刷新地圖
175 }
176
177 //沒吃到食物
178 inline void FAILURE()
179 {
180 map[snake[tail].x][snake[tail].y] = ' '; //蛇尾原來的位置變成“ ”
181 tail = (tail + 1) % 400; //蛇尾下標加1
182 map[Next.x][Next.y] = '@'; //蛇頭位置變化
183 map[snake[head].x][snake[head].y] = 'O'; //原蛇頭位置變化為蛇身
184 head = (head + 1) % 400; //蛇頭下標加1
185 snake[head].x = Next.x; //蛇頭下標變化
186 snake[head].y = Next.y;
187 Update(map, grade, length, autotime); //刷新地圖
188 }
189
190 //main函數
191 int main()
192 {
193 system("color F0");
194 init(); //初始化
195 hello(); //歡迎界面
196 while (1)
197 {
198 if (GO()) //預前進
199 {
200 if (Next.x == food.x&&Next.y == food.y)
201 EAT(); //吃到食物
202 else
203 FAILURE(); //沒吃到食物
204 }
205 else
206 return 0; //失敗或者勝利,游戲結束
207 }
208 return 0;
209 }
210 //
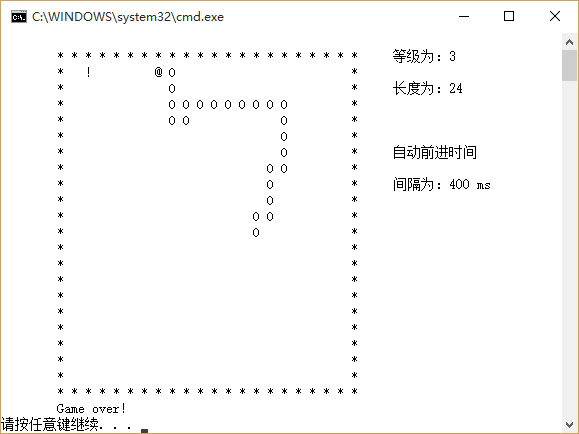