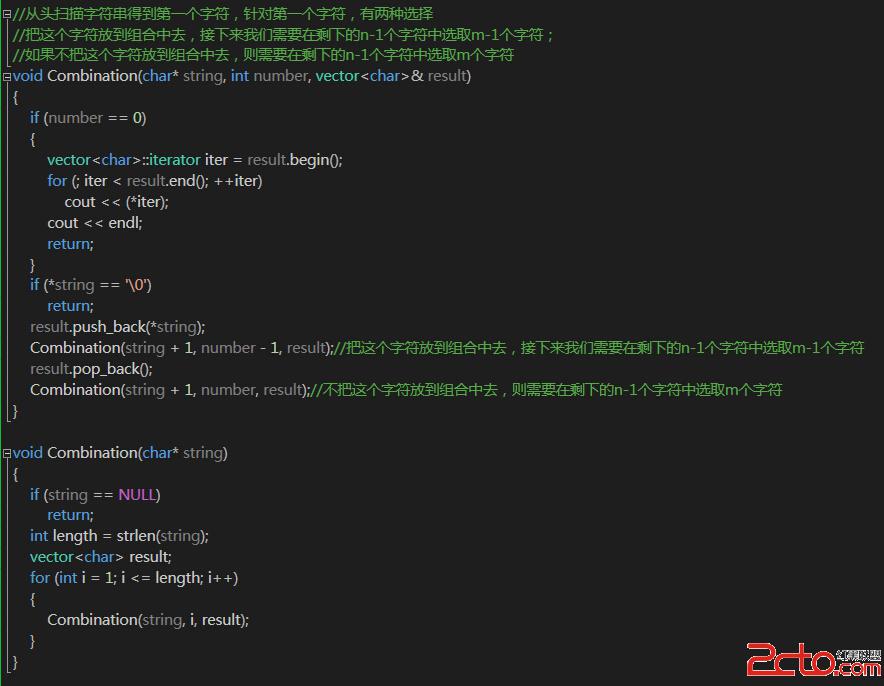
#include
#include
#include
using namespace std;
//從頭掃描字符串得到第一個字符,針對第一個字符,有兩種選擇
//把這個字符放到組合中去,接下來我們需要在剩下的n-1個字符中選取m-1個字符;
//如果不把這個字符放到組合中去,則需要在剩下的n-1個字符中選取m個字符
void Combination(char* string, int number, vector& result)
{
if (number == 0)
{
vector::iterator iter = result.begin();
for (; iter < result.end(); ++iter)
cout << (*iter);
cout << endl;
return;
}
if (*string == '\0')
return;
result.push_back(*string);
Combination(string + 1, number - 1, result);//把這個字符放到組合中去,接下來我們需要在剩下的n-1個字符中選取m-1個字符
result.pop_back();
Combination(string + 1, number, result);//不把這個字符放到組合中去,則需要在剩下的n-1個字符中選取m個字符
}
void Combination(char* string)
{
if (string == NULL)
return;
int length = strlen(string);
vector result;
for (int i = 1; i <= length; i++)
{
Combination(string, i, result);
}
}
int main()
{
char s[] = "abc";
Combination(s);
system("pause");
return 0;
}