C之五子棋,五子棋
1 #include <stdio.h>
2 #include <stdlib.h>
3
4 #define N 15
5
6 int chessboard[N + 1][N + 1] = { 0 };
7
8 int whoseTurn = 0;
9
10 void initGame(void);
11 void printChessboard(void);
12 void playChess(void);
13 int judge(int, int);
14
15 int main(void)
16 {
17 initGame();
18
19 while (1)
20 {
21 whoseTurn++;
22
23 playChess();
24 }
25
26 return 0;
27 }
28
29 void initGame(void)
30 {
31 char c;
32
33 printf("Please input \'y\' to enter the game:");
34 c = getchar();
35 if ('y' != c && 'Y' != c)
36 exit(0);
37
38 system("cls");
39 printChessboard();
40 }
41
42 void printChessboard(void)
43 {
44 int i, j;
45
46 for (i = 0; i <= N; i++)
47 {
48 for (j = 0; j <= N; j++)
49 {
50 if (0 == i)
51 printf("%3d", j);
52 else if (j == 0)
53 printf("%3d", i);
54 else if (1 == chessboard[i][j])
55 printf(" O");
56 else if (2 == chessboard[i][j])
57 printf(" X");
58 else
59 printf(" *");
60 }
61 printf("\n");
62 }
63 }
64
65 void playChess(void)
66 {
67 int i, j, winner;
68
69 if (1 == whoseTurn % 2)
70 {
71 printf("Turn to player 1, please input the position:");
72 scanf("%d %d", &i, &j);
73
74 while (chessboard[i][j] != 0)
75 {
76 printf("This position has been occupied, please input the position again:");
77 scanf("%d %d", &i, &j);
78 }
79
80 chessboard[i][j] = 1;
81 }
82 else
83 {
84 printf("Turn to player 1, please input the position:");
85 scanf("%d %d", &i, &j);
86
87 while (chessboard[i][j] != 0)
88 {
89 printf("This position has been occupied, please input the position again:");
90 scanf("%d %d", &i, &j);
91 }
92
93 chessboard[i][j] = 2;
94 }
95
96 system("cls");
97 printChessboard();
98
99 if (judge(i, j))
100 {
101 if (1 == whoseTurn % 2)
102 {
103 printf("Winner is player 1!\n");
104 exit(0);
105 }
106 else
107 {
108 printf("Winner is player 2!\n");
109 exit(0);
110 }
111 }
112 }
113
114 int judge(int x, int y)
115 {
116 int i, j;
117 int t = 2 - whoseTurn % 2;
118
119 for (i = x - 4, j = y; i <= x; i++)
120 {
121 if (i >= 1 && i <= N - 4 && t == chessboard[i][j] && t == chessboard[i + 1][j] && t == chessboard[i + 2][j] && t == chessboard[i + 3][j] && t == chessboard[i + 4][j])
122 return 1;
123 }
124 for (i = x, j = y - 4; j <= y; j++)
125 {
126 if (j >= 1 && j <= N - 4 && t == chessboard[i][j] && t == chessboard[i][j + 1] && t == chessboard[i][j + 1] && t == chessboard[i][j + 3] && t == chessboard[i][j + 4])
127 return 1;
128 }
129 for (i = x - 4, j = y - 4; i <= x, j <= y; i++, j++)
130 {
131 if (i >= 1 && i <= N - 4 && j >= 1 && j <= N - 4 && t == chessboard[i][j] && t == chessboard[i + 1][j + 1] && t == chessboard[i + 2][j + 2] && t == chessboard[i + 3][j + 3] && t == chessboard[i + 4][j + 4])
132 return 1;
133 }
134 for (i = x + 4, j = y - 4; i >= 1, j <= y; i--, j++)
135 {
136 if (i >= 1 && i <= N - 4 && j >= 1 && j <= N - 4 && t == chessboard[i][j] && t == chessboard[i - 1][j + 1] && t == chessboard[i - 2][j + 2] && t == chessboard[i - 3][j + 3] && t == chessboard[i - 4][j + 4])
137 return 1;
138 }
139
140 return 0;
141 }
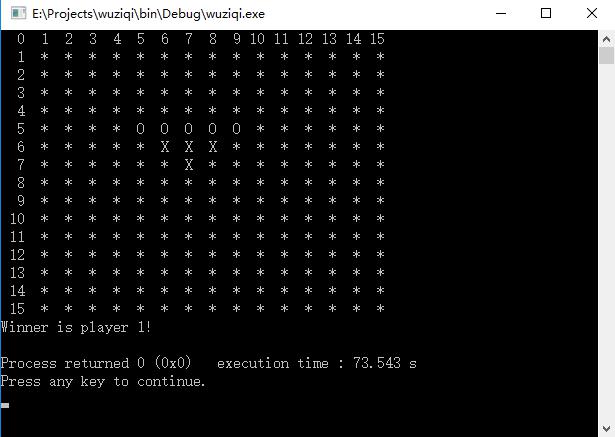