WinForm 窗體應用程序 (初步)之二,winform窗體
現在,我們來了解一些基本控件。控件是放置在工具箱裡的,你可以在界面的左側或者通過菜單欄的視圖選項找到它。
(1)Label 控件 這是一個用於放置文字的控件,因為你不能在窗體上直接輸入文字。
(2)TextBox 文本框
(3)Button 按鈕
(4)CheckBox 復選框
(5)Panel 分組容器,類似於HTML中的div
(6)PictureBox 圖片框
(7)WebBrowser 它可以允許用戶在窗體內浏覽網頁,可用於制作浏覽器
下面附上筆者自制的一個拼圖游戲及代碼文件: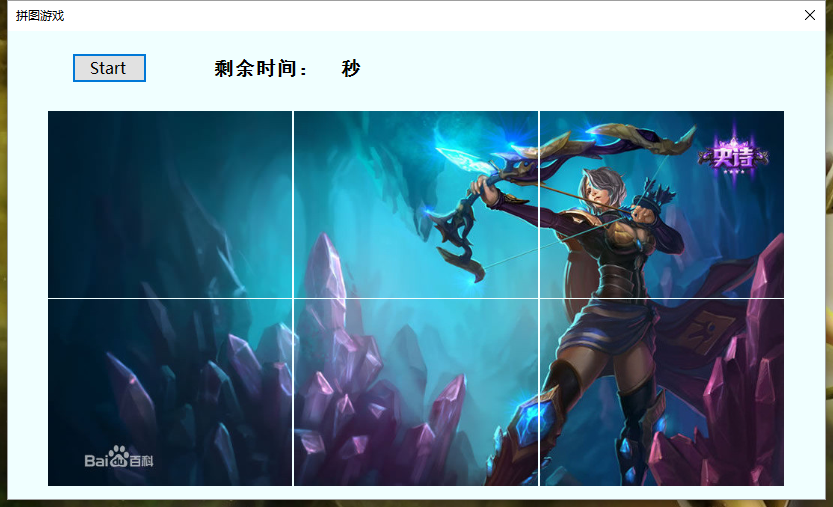

![]()
1 using System;
2 using System.Collections;
3 using System.Collections.Generic;
4 using System.ComponentModel;
5 using System.Data;
6 using System.Drawing;
7 using System.Linq;
8 using System.Text;
9 using System.Threading.Tasks;
10 using System.Windows.Forms;
11
12 namespace WindowsFormsApplication1
13 {
14 public partial class Form1 : Form
15 {
16 public Form1()
17 {
18 InitializeComponent();
19 ImgList = null;
20 }
21 #region 定義字段
22 List<Image> _imgList;
23 /// <summary>
24 /// 定義屬性
25 /// </summary>
26 public List<Image> ImgList
27 {
28 get { return _imgList; }
29 set
30 {
31 _imgList = new List<Image>();
32 _imgList.Add(pictureBox1.BackgroundImage);
33 _imgList.Add(pictureBox2.BackgroundImage);
34 _imgList.Add(pictureBox3.BackgroundImage);
35 _imgList.Add(pictureBox4.BackgroundImage);
36 _imgList.Add(pictureBox5.BackgroundImage);
37 _imgList.Add(pictureBox6.BackgroundImage);
38 }
39 }
40 #endregion
41 #region 開始按鈕
42 private void button1_Click(object sender, EventArgs e)
43 {
44 //隨機6個不同的數
45 Random rd = new Random();
46 int[] x = new int[6];
47 for (int i = 0; i < 6; i++)
48 {
49 x[i] = rd.Next(0, 6);
50 for (int j = 0; j < i; j++)
51 {
52 if (x[i] == x[j])
53 {
54 i--;
55 break;
56 }
57 }
58 }
59 //重新設置圖像
60 pictureBox1.BackgroundImage = ImgList[x[0]];
61 pictureBox2.BackgroundImage = ImgList[x[1]];
62 pictureBox3.BackgroundImage = ImgList[x[2]];
63 pictureBox4.BackgroundImage = ImgList[x[3]];
64 pictureBox5.BackgroundImage = ImgList[x[4]];
65 pictureBox6.BackgroundImage = ImgList[x[5]];
66 //倒計時開始,並允許玩家操作
67 time = 5;
68 label2.Text="5";
69 timer1.Start();
70 pictureBox1.Enabled = true;
71 pictureBox2.Enabled = true;
72 pictureBox3.Enabled = true;
73 pictureBox4.Enabled = true;
74 pictureBox5.Enabled = true;
75 pictureBox6.Enabled = true;
76 }
77 #endregion
78 #region 玩家操作
79 //定義匹配變量
80 int match = 0;
81 //存儲上一張圖片
82 PictureBox lpb = new PictureBox();
83 //響應用戶操作
84 private void pictureBox1_Click(object sender, EventArgs e)
85 {
86 PictureBox pb = sender as PictureBox;
87 //截取Name的最後一位作為唯一標識
88 int n = int.Parse(pb.Name.Substring(10, 1));
89 //判斷是否已經正確歸位,如果沒有正確歸位
90 if (pb.BackgroundImage != ImgList[n - 1])
91 {
92 //重置參數
93 if (match == 2)
94 {
95 match = 0;
96 }
97 //交換背景圖片
98 if (match == 1)
99 {
100 Image img = pb.BackgroundImage;
101 pb.BackgroundImage = lpb.BackgroundImage;
102 lpb.BackgroundImage = img;
103 //判斷是否全部歸位
104 if (pictureBox1.BackgroundImage == ImgList[0] && pictureBox2.BackgroundImage == ImgList[1] && pictureBox3.BackgroundImage == ImgList[2] && pictureBox4.BackgroundImage == ImgList[3] && pictureBox5.BackgroundImage == ImgList[4] && pictureBox6.BackgroundImage == ImgList[5])
105 {
106 timer1.Stop();
107 MessageBox.Show("恭喜您,順利過關!");
108 }
109 }
110 lpb = pb;
111 match++;
112 }
113 }
114 #endregion
115 #region 計時功能
116 int time = 5;
117 private void timer1_Tick(object sender, EventArgs e)
118 {
119 if (time > 0)
120 {
121 time--;
122 label2.Text = time.ToString();
123 }
124 else
125 {
126 //停止計時,並禁止玩家操作
127 timer1.Stop();
128 pictureBox1.Enabled = false;
129 pictureBox2.Enabled = false;
130 pictureBox3.Enabled = false;
131 pictureBox4.Enabled = false;
132 pictureBox5.Enabled = false;
133 pictureBox6.Enabled = false;
134 MessageBox.Show("很遺憾,游戲失敗!");
135 }
136 }
137 #endregion
138
139
140
141 }
142 }
View Code