一.設計時效果

二.運行時效果
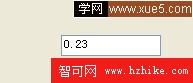
三.簡單談談實現思路
該控件實現了控制粘貼,控制輸入有效精度,負數控制,整數控制等等操作,將為快速開發提供方便.
四.源代碼

using System;

using System.Collections.Generic;

using System.ComponentModel;

using System.Drawing;

using System.Data;

using System.Text;

using System.Windows.Forms;


namespace JcsExpLibary.Numeric_Textbox


...{

public partial class JcsInputNum : TextBox


...{

private bool _isreadonly = true ; //是否允許回車代替TAB

private bool _isintegral = false ;//是否整數輸入

private bool _EnPaste = true ;//是否允許粘貼

private bool _EnContextMenu = true ; //是否允許現實右鍵菜單

private int _PointNumber = -1 ;//為-1時允許輸入任意位小數

private bool _isnegativenumber = false; //是否允許輸入負數

public delegate void InputNumberTextBoxEvent();

public event InputNumberTextBoxEvent PasteEvent;

public JcsInputNum()


...{

InitializeComponent();

}


/**//// <summary>

/// 是否只讀

/// </summary>

[Category("JCS屬性"),Description("是否只讀。")]

public bool Isreadonly


...{


get ...{ return this._isreadonly; }


set ...{ this._isreadonly = value; }

}


/**//// <summary>

/// 是否是整數

/// </summary>

[Category("JCS屬性"), Description("是否整數。")]

public bool isintegral


...{


get ...{ return this._isintegral; }


set ...{ _isintegral = value; }

}


/**//// <summary>

/// 是否允許輸入負數

/// </summary>

[Category("JCS屬性"), Description("是否允許輸入負數。")]

public bool IsNegativeNumber


...{


get ...{ return _isnegativenumber; }


set ...{ _isnegativenumber = value; }

}


/**//// <summary>

/// 精度位數控制(即:允許輸入幾位小數控制)

/// </summary>

[Category("JCS屬性"), Description("精度位數控制,-1時允許輸入任意位小數。")]

public int PointNumber


...{


get ...{ return _PointNumber; }


set ...{ this._PointNumber = value; }

}

[Category("JCS屬性"), Description("是否允許粘貼。")]

public bool EnablePaste


...{


get ...{ return _EnPaste; }

set


...{

_EnPaste = value;

this.Invalidate();

}

}


/**//// <summary>

&nbs ///

/// </summary>

[Category("JCS屬性"), Description("是否右鍵菜單。")]

public bool EnableContextMenu


...{



get ...{ return _EnContextMenu; }

set


...{

_EnContextMenu = value;

this.Invalidate();

}

}




"private and overrides function"#region "private and overrides function"


/**//// <summary>

/// 檢驗輸入

/// </summary>

/// <param name="e"></param>

/// <param name="KeyAsc"></param>

/// <param name="CurPos"></param>

private void ValidNumeric(System.Windows.Forms.KeyPressEventArgs e, int KeyAsc, int CurPos)


...{

switch (KeyAsc)


...{

case 8:

case 13:

// Backspace, Enter

break;

// Allow entry

case 48:

case 49:

case 50:

case 51:

case 52:

case 53:

case 54:

case 55:

case 56:

case 57:

//0-9 modifIEd by jcs 2007-02-28 針對數字小數點精度的限制做了調整

// Allow entry

if (this._PointNumber != -1)


...{

int index = this.Text.IndexOf(".");

if (index != -1)


...{

int startindex = this.SelectionStart;

if (startindex >= index)


...{

//如果光標位置在小數點右側,則要判斷是否已經輸入了有效位的數字

int nUMLength = this.Text.Substring(index+1).Length + 1;

if (nUMLength > this._PointNumber & this.SelectionLength == 0)


...{

e.Handled = true;

return;

}

}

else


...{

//如果光標位置在小數點左側,則允許輸入

}

}

}


break;

case 46:

//小數點

//是否整數

if (this._isintegral)


...{

e.Handled = true;

return; //

}


//小數點多於1個時

if (this.Text.IndexOf( ".") != -1)


...{

e.Handled = true;

}

else


...{

&n //在起始位置時

if (CurPos == 0)


...{

this.Text = "0" + this.Text;

//Add a zero before it

this.SelectionStart = 1;

//Set original cursor position光標在小數點後的位置

}


else if (CurPos == 1 & this.Text.StartsWith("-"))


...{

//負數

this.Text = "-0." + this.Text.Substring(1);

//Add zero before decimal連接小數點後的內容

this.SelectionStart = 3;

e.Handled = true;

}

else


...{

this.Text = this.Text + ".00";

//Append Decimal and two zero''s 增添精確小數位數

this.SelectionStart = CurPos + 1;

this.SelectionLength = 2;

//Highlight the zero''s

e.Handled = true;

}

}


break;

case 45:

//負號

if (!this._isnegativenumber)


...{

e.Handled = true;

return;

}

else


...{

if (CurPos != 0)


...{

//開始位置

e.Handled = true;

return;

}

}


break;

default:

e.Handled = true;


break;

}

}

//Validate Input data for Numeric

&nbs protected override void OnKeyPress(System.Windows.Forms.KeyPressEventArgs e)


...{


int KeyAsc;

//Ascii code of character

int CurPos;

//Cursor position


KeyAsc = Convert.ToInt32(e.KeyChar);

CurPos = this.SelectionStart;


ValidNumeric(e, KeyAsc, CurPos);


}

protected override void OnKeyDown(System.Windows.Forms.KeyEventArgs e)


...{

if (_isreadonly)


...{

if (e.KeyCode == System.Windows.Forms.Keys.Enter)


...{

SendKeys.Send("{TAB}");

}

}

}


protected override void OnGotFocus(System.EventArgs e)


...{

this.Select();

this.SelectAll();

}

protected override void WndProc(ref System.Windows.Forms.Message m)


...{

switch (m.Msg)


...{

case 770:

//paste

if (!_EnPaste)

return;

IDataObject iData = Clipboard.GetDataObject();

if (iData.GetDataPresent(DataFormats.Text))


...{

if (!IsNumeric(iData.ToString()))


...{

return ;

}

}

else


...{

return ;

}

if (PasteEvent != null)


...{

PasteEvent();

}

break;

case 123:

//contextmenu

if (!_EnContextMenu)

return;

break;

case 256:

case 260:

//按下一個鍵,WM_KEYDOWN;WM_SYSKEYDOWN

//switch (m.WParam.ToInt32)

//{

// case Keys.Enter:

// SendKeys.Send("{tab}");

// break;

// case Keys.Left:


// return;

// case Keys.Right:


// return;

// case Keys.Up:


// return;

// case Keys.Down:


// return;

// case Keys.Shift + Keys.Tab:

// return;

// case Keys.Tab:

// break;


//}

break;


}

base.WndProc(ref m);

}

public bool IsNumeric(string str)


...{

char[] ch = new char[str.Length];

ch = str.ToCharArray();

for (int i = 0; i < ch.Length; i++)


...{

if (ch[i] < 48 || ch[i] > 57)

return false;

}

return true;

}


#endregion



}


}