:

public partial class RichTextBox : Form


...{

public RichTextBox()


...{

InitializeComponent();

txtSize.KeyPress += new KeyPressEventHandler(txtSize_KeyPress);

txtSize.Validating += new CancelEventHandler(txtSize_Validating);

rtpText.LinkClicked += new LinkClickedEventHandler(rtpText_LinkClicked);

}


void rtpText_LinkClicked(object sender, LinkClickedEventArgs e)


...{

System.Diagnostics.Process.Start(e.LinkText);

}


void txtSize_Validating(object sender, CancelEventArgs e)


...{

= (TextBox)sender;

ApplyTextSize(txt.Text);

rtpText.Focus();

}


void txtSize_KeyPress(object sender, KeyPressEventArgs e)


...{

if ((e.KeyChar < 48 || e.KeyChar > 57) && e.KeyChar != 8 && e.KeyChar != 13)


...{

e.Handled = true;

}

else if (e.KeyChar == 13)


...{

TextBox txt = (TextBox)sender;

if (txt.Text.Length > 0)

ApplyTextSize(txt.Text);

e.Handled = true;

rtpText.Focus();

}
}

void ApplyTextSize(string txt)


...{

if (txtSize.Text.Trim().Length > 0)


...{

float newSize = Convert.ToSingle(txtSize.Text.Trim());

FontFamily currentFontFamily;

Font newFont;

currentFontFamily = rtpText.SelectionFont.FontFamily;

newFont = new Font(currentFontFamily, newSize);

rtpText.SelectionFont = newFont;

}

}

private void btnBold_Click(object sender, EventArgs e)


...{

try

Font oldFont;

Font newFont;

oldFont = rtpText.SelectionFont;

if (oldFont.Bold == true)

newFont = new Font(oldFont, oldFont.Style & ~FontStyle.Bold);

else newFont = new Font(oldFont, oldFont.Style | FontStyle.Bold);

rtpText.SelectionFont = newFont;

this.rtpText.Focus();

}

catch (Exception ex)


...{

MessageBox.Show(ex.ToString());

}

}


private void btnItalic_Click(object sender, EventArgs e)


...{

Font oldFont;

Font newFont;

oldFont = rtpText.SelectionFont;

.Italic == true)

newFont = new Font(oldFont, oldFont.Style & ~FontStyle.Italic);

else newFont = new Font(oldFont, oldFont.Style | FontStyle.Italic);

rtpText.SelectionFont = newFont;

this.rtpText.Focus();

}


private void btnUnderline_Click(object sender, EventArgs e)


...{

Font oldFont;

Font newFont;

oldFont = rtpText.SelectionFont;

if (oldFont.Underline == true)

newFont = new Font(oldFont, oldFont.Style & ~FontStyle.Underline);

else newFont = new Font(oldFont, oldFont.Style | FontStyle.Underline);

rtpText.SelectionFont = newFont;

this.rtpText.Focus();

}


private void btnCenter_Click(object sender, EventArgs e)


...{

if (this.rtpText.SelectionAlignment == HorizontalAlignment.Center)
rtpText.SelectionAlignment = HorizontalAlignment.Left;

else rtpText.SelectionAlignment = HorizontalAlignment.Center;

rtpText.Focus();

}


private void btnSave_Click(object sender, EventArgs e)


...{

//string FilePath = Application.StartupPath + @"Test.rtf";

//bool exist = File.Exists(FilePath);

//if (exist == false)

//{

// Directory.CreateDirectory(Application.StartupPath);

// FileStream fs = new FileStream(FilePath, FileMode.Create);

// fs.Close();

//}

try


...{

rtpText.SaveFile("../../Test.rtf");

}

catch (Exception err)



MessageBox.Show(err.Message);

}

}


private void btnLoad_Click(object sender, EventArgs e)


...{

try


...{

rtpText.LoadFile("../../Test.rtf");

}

catch (System.IO.FileNotFoundException)


...{

MessageBox.Show("沒找到相關文件");

}

}

private void btnStart_Click(object sender, EventArgs e)


System.Diagnostics.Process.Start(Application.StartupPath + @"垃圾清理.bat");

}

}
運行效果圖:
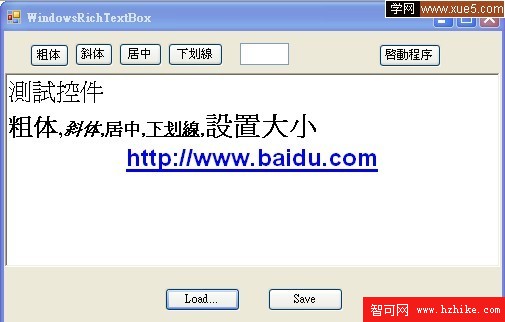