In the usual games , We are Excel After the data is written in it , You need to click the button to rank , Xiao Wang felt a little annoyed , As a powerful programming language Python, Can't you ? The answer is , Certainly. !
Project description : After program running , Prompt the user to have an option menu , Users can enter scores according to the menu , Easy and convenient , Check at any time !
Related to knowledge : Tuple list , Conditional statements , loop , character string , Simple algorithm , Dictionaries , function
Tuple algorithm
# -*- coding : utf-8 -*-
# @Time : 2020/8/8
# @author : Wang Xiaowang
# @Software : PyCharm
# @CSDN : https://blog.csdn.net/weixin_47723732
scores = []
choice = None
print('''
Score entry applet
0 - End procedure
1 - Enter the name score and enter
2 - Print the results after ranking
3 - Find grades for specific students
4 - Delete grades for specific students
''')
while choice != "0":
choice = input("Choice: ")
if choice == "0":
print(" End procedure !")
elif choice == "1":
name = input(" Enter a name : ")
score = int(input(" Enter grades : "))
listing = (score, name)
scores.append(listing)
scores.sort(reverse=True)
elif choice == "2":
print(" Results ranking ")
print(" full name \t achievement ")
for listing in scores:
score, name = listing
print(name, "\t", score)
elif choice == "3":
Name = input(" Enter the name you want to find :")
for each in scores:
if each[1] == Name:
score ,name=each
print(" full name \t achievement ")
print(name, "\t", score )
elif choice == "4":
Name = input(" Enter the name of the grade you want to delete : ")
for each in scores:
if each[1] == Name:
scores.remove(each)
else:
print(" The choice you entered is wrong , Please check !")
input("\n\nPress the enter key to exit.")
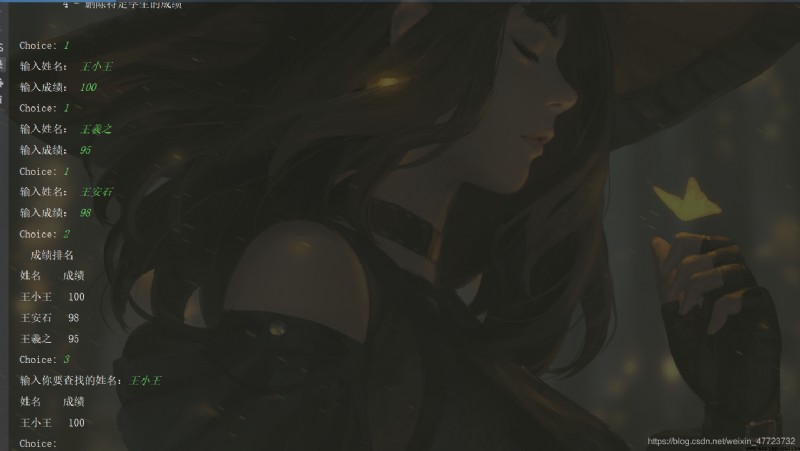
Dictionary algorithm
# -*- coding : utf-8 -*-
# @Time : 2020/8/8
# @author : Wang Xiaowang
# @Software : PyCharm
# @CSDN : https://blog.csdn.net/weixin_47723732
# All student data is saved to a list , Each student's information is represented in a dictionary [{},{}]
records = []
def print_menu():
"""
Print out the entire menu
:return:
"""
print("""
Student information system
0 - sign out
1 - Show all student information
2 - Add student information
3 - Search for student information
4 - Delete student information
5 - Sort
""")
def user_choice():
"""
Get user input , And make sure that the input data is in 0-4 Between
:return: Returns the legal user selection
"""
choice = input(" Please select (0~5):")
# Make sure the user's choice is in the 0~4
while int(choice) > 5 or int(choice) < 0:
choice = input(" Please reselect (0~5):")
return choice
def add_record():
"""
Add user data
:return:
"""
name = input(' Please enter the name of the student :')
while name == '':
name = input(' Please enter the name of the student :')
# Determine whether the student information has been entered
for info in records:
if info['name'] == name:
print(" The student already exists ")
break
else:
score = float(input(' Please enter the grade (0~100):'))
while score < 0 or score > 100:
score = float(input(' Please enter the grade (0~100):'))
info = {
'name': name,
'score': score
}
records.append(info)
def display_records():
"""
Display data
:return:
"""
print(" All student information ")
for info in records:
print(" full name :", info['name'], ' achievement :', info['score'])
def search_record():
"""
Look up the information according to the student's name , And output the students' scores
:return:
"""
name = input(' Please enter the name of the student :')
while name == '':
name = input(' Please enter the name of the student :')
for info in records:
if info['name'] == name:
print(" Student achievement is :", info['score'])
break
else:
print(" There is no such student ")
def del_record():
"""
Delete data according to student name
:return:
"""
name = input(' Please enter the name of the student :')
while name == '':
name = input(' Please enter the name of the student :')
for info in records:
if info['name'] == name:
records.remove(info)
break
else:
print(" There is no such user ")
choice = None
while choice != "0":
# Print menu
print_menu()
# Get user input
choice = user_choice()
# Use conditional branching to determine various options
if choice == '1':
# Display the data
display_records()
elif choice == '2':
# Add a student data
add_record()
elif choice == '3':
# find information
search_record()
elif choice == '4':
# Delete data
del_record()
elif choice == '5':
records.sort(key=lambda item: item['score'])
elif choice == '0':
# Exit procedure
print(' Welcome to login this system next time ')
else:
print(' Wrong choice !!!')
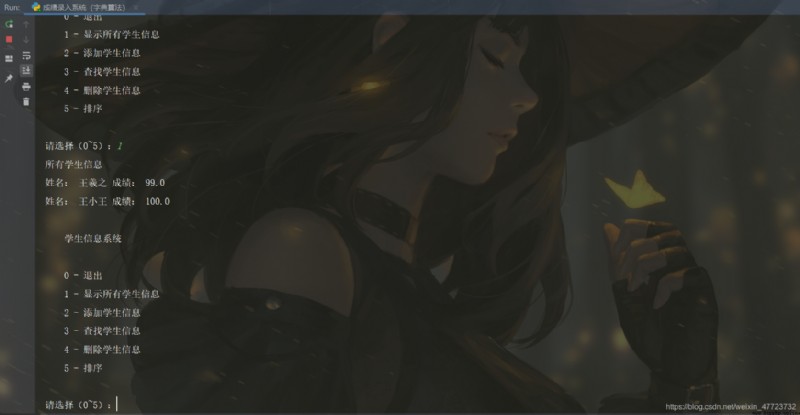
You can try it yourself !