第十八章 springboot + thymeleaf,springbootthymeleaf
代碼結構:
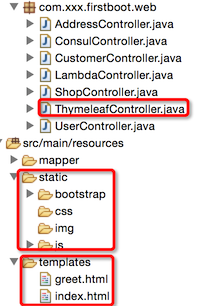
1、ThymeleafController

![]()
1 package com.xxx.firstboot.web;
2
3 import org.springframework.stereotype.Controller;
4 import org.springframework.ui.Model;
5 import org.springframework.web.bind.annotation.RequestMapping;
6 import org.springframework.web.bind.annotation.RequestMethod;
7 import org.springframework.web.bind.annotation.RequestParam;
8 import org.springframework.web.bind.annotation.ResponseBody;
9
10 import io.swagger.annotations.Api;
11 import io.swagger.annotations.ApiOperation;
12
13 @Api("測試Thymeleaf和devtools")
14 @Controller
15 @RequestMapping("/thymeleaf")
16 public class ThymeleafController {
17
18 @ApiOperation("第一個thymeleaf程序")
19 @RequestMapping(value = "/greeting", method = RequestMethod.GET)
20 public String greeting(@RequestParam(name = "name", required = false, defaultValue = "world") String name,
21 Model model) {
22 model.addAttribute("xname", name);
23 return "index";
24 }
25
26 @ApiOperation("thymeleaf ajax")
27 @ResponseBody
28 @RequestMapping(value = "/ajax", method = RequestMethod.GET)
29 public String ajax(@RequestParam("username") String username) {
30 return username;
31 }
32
33 }
View Code
說明:
- 第一個是springMVC經典返回形式modelAndView
- 第二個是ajax返回形式
2、index.html
1 <!DOCTYPE HTML>
2 <html xmlns:th="http://www.thymeleaf.org">
3 <head>
4 <title>index</title>
5 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
6
7 <link th:href="@{/bootstrap/css/bootstrap.min.css}" rel="stylesheet"/>
8 <link th:href="@{/bootstrap/css/bootstrap-theme.min.css}" rel="stylesheet" />
9 </head>
10 <body>
11 <div class="panel panel-primary">
12 <div class="panel-heading">hello</div>
13 <div class="panel-body" th:text="${xname}"></div>
14 </div>
15 <div class="panel panel-warning">
16 <div class="panel-heading">hello</div>
17 <div id="usernamediv" class="panel-body"></div>
18 </div>
19 <script type="text/javascript" th:src="@{/js/jquery-1.11.1.js}"></script>
20 <script type="text/javascript" th:src="@{/bootstrap/js/bootstrap.min.js}"></script>
21 <script th:inline="javascript">
22 $(function(){
23 $.ajax({
24 url:"/thymeleaf/ajax",
25 data:{
26 username:"xxx"
27 },
28 type:"get",
29 dataType:"text",
30 success:function(text){
31 alert(text);
32 $("#usernamediv").text(text);
33 }
34 });
35 });
36 </script>
37 </body>
38 </html>
說明:
- 引入外界靜態資源的方式@{/xxx},默認的靜態資源的根是"static"
- ajax的返回類型dataType要選好(一般就是"text"/"json")
- ajax的請求方法類型type要與controller相同,否則拋出405錯誤
文檔:
- thymeleaf的其他配置查看:《springboot實戰》、http://www.thymeleaf.org/doc/tutorials/2.1/usingthymeleaf.html
- bootstrap:http://www.bootcss.com/