第四章 springboot + swagger,springbootswagger
注:本文參考自
http://www.jianshu.com/p/0465a2b837d2
swagger用於定義API文檔。
好處:
- 前後端分離開發
- API文檔非常明確
- 測試的時候不需要再使用URL輸入浏覽器的方式來訪問Controller
- 傳統的輸入URL的測試方式對於post請求的傳參比較麻煩(當然,可以使用postman這樣的浏覽器插件)
- spring-boot與swagger的集成簡單的一逼
1、項目結構
和上一節一樣,沒有改變。
2、pom.xml
引入了兩個jar。

![]()
1 <dependency>
2 <groupId>io.springfox</groupId>
3 <artifactId>springfox-swagger2</artifactId>
4 <version>2.2.2</version>
5 </dependency>
6 <dependency>
7 <groupId>io.springfox</groupId>
8 <artifactId>springfox-swagger-ui</artifactId>
9 <version>2.2.2</version>
10 </dependency>
View Code
3、Application.java

![]()
1 package com.xxx.firstboot;
2
3 import org.springframework.boot.SpringApplication;
4 import org.springframework.boot.autoconfigure.SpringBootApplication;
5
6 import springfox.documentation.swagger2.annotations.EnableSwagger2;
7
8 @SpringBootApplication //same as @Configuration+@EnableAutoConfiguration+@ComponentScan
9 @EnableSwagger2 //啟動swagger注解
10 public class Application {
11
12 public static void main(String[] args) {
13 SpringApplication.run(Application.class, args);
14 }
15
16 }
View Code
說明:
- 引入了一個注解@EnableSwagger2來啟動swagger注解。(啟動該注解使得用在controller中的swagger注解生效,覆蓋的范圍由@ComponentScan的配置來指定,這裡默認指定為根路徑"com.xxx.firstboot"下的所有controller)
4、UserController.java

![]()
1 package com.xxx.firstboot.web;
2
3 import org.springframework.beans.factory.annotation.Autowired;
4 import org.springframework.web.bind.annotation.RequestHeader;
5 import org.springframework.web.bind.annotation.RequestMapping;
6 import org.springframework.web.bind.annotation.RequestMethod;
7 import org.springframework.web.bind.annotation.RequestParam;
8 import org.springframework.web.bind.annotation.RestController;
9
10 import com.xxx.firstboot.domain.User;
11 import com.xxx.firstboot.service.UserService;
12
13 import io.swagger.annotations.Api;
14 import io.swagger.annotations.ApiImplicitParam;
15 import io.swagger.annotations.ApiImplicitParams;
16 import io.swagger.annotations.ApiOperation;
17 import io.swagger.annotations.ApiResponse;
18 import io.swagger.annotations.ApiResponses;
19
20 @RestController
21 @RequestMapping("/user")
22 @Api("userController相關api")
23 public class UserController {
24
25 @Autowired
26 private UserService userService;
27
28 // @Autowired
29 // private MyRedisTemplate myRedisTemplate;
30
31 @ApiOperation("獲取用戶信息")
32 @ApiImplicitParams({
33 @ApiImplicitParam(paramType="header",name="username",dataType="String",required=true,value="用戶的姓名",defaultValue="zhaojigang"),
34 @ApiImplicitParam(paramType="query",name="password",dataType="String",required=true,value="用戶的密碼",defaultValue="wangna")
35 })
36 @ApiResponses({
37 @ApiResponse(code=400,message="請求參數沒填好"),
38 @ApiResponse(code=404,message="請求路徑沒有或頁面跳轉路徑不對")
39 })
40 @RequestMapping(value="/getUser",method=RequestMethod.GET)
41 public User getUser(@RequestHeader("username") String username, @RequestParam("password") String password) {
42 return userService.getUser(username,password);
43 }
44
45 // @RequestMapping("/testJedisCluster")
46 // public User testJedisCluster(@RequestParam("username") String username){
47 // String value = myRedisTemplate.get(MyConstants.USER_FORWARD_CACHE_PREFIX, username);
48 // if(StringUtils.isBlank(value)){
49 // myRedisTemplate.set(MyConstants.USER_FORWARD_CACHE_PREFIX, username, JSON.toJSONString(getUser()));
50 // return null;
51 // }
52 // return JSON.parseObject(value, User.class);
53 // }
54
55 }
View Code
說明:
- @Api:用在類上,說明該類的作用
- @ApiOperation:用在方法上,說明方法的作用
- @ApiImplicitParams:用在方法上包含一組參數說明
- @ApiImplicitParam:用在@ApiImplicitParams注解中,指定一個請求參數的各個方面
- paramType:參數放在哪個地方
- header-->請求參數的獲取:@RequestHeader
- query-->請求參數的獲取:@RequestParam
- path(用於restful接口)-->請求參數的獲取:@PathVariable
- body(不常用)
- form(不常用)
- name:參數名
- dataType:參數類型
- required:參數是否必須傳
- value:參數的意思
- defaultValue:參數的默認值
- @ApiResponses:用於表示一組響應
- @ApiResponse:用在@ApiResponses中,一般用於表達一個錯誤的響應信息
- code:數字,例如400
- message:信息,例如"請求參數沒填好"
- response:拋出異常的類
以上這些就是最常用的幾個注解了。
具體其他的注解,查看:
https://github.com/swagger-api/swagger-core/wiki/Annotations#apimodel
測試:
啟動服務,浏覽器輸入"http://localhost:8080/swagger-ui.html"
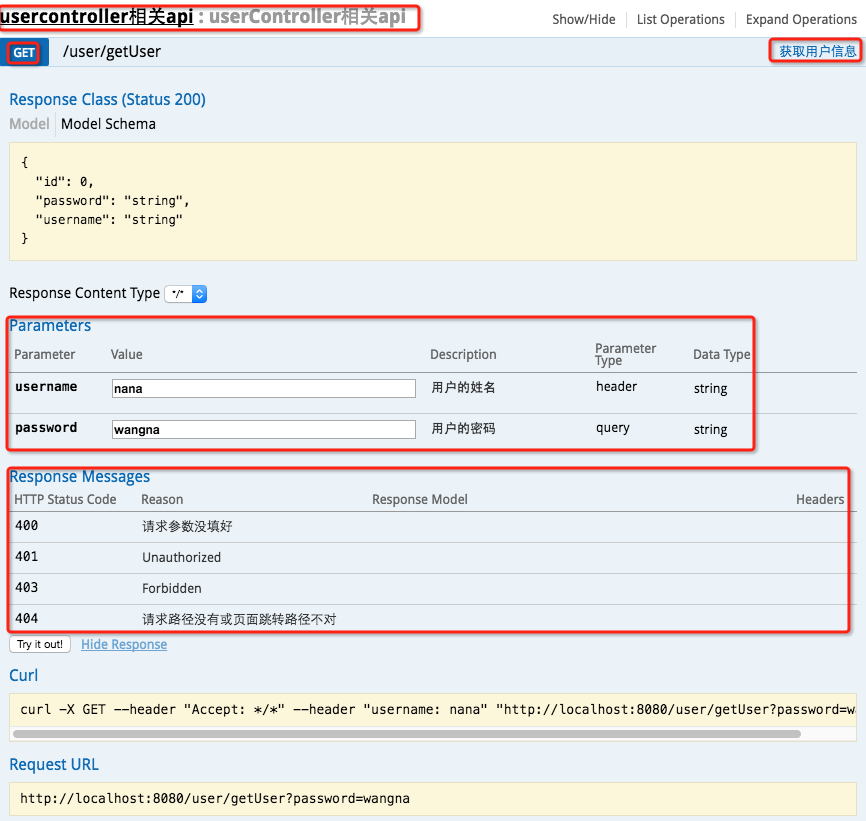
最上邊一個紅框:@Api
GET紅框:method=RequestMethod.GET
右邊紅框:@ApiOperation
parameter紅框:@ApiImplicitParams系列注解
response messages紅框:@ApiResponses系列注解
輸入參數後,點擊"try it out!",查看響應內容:
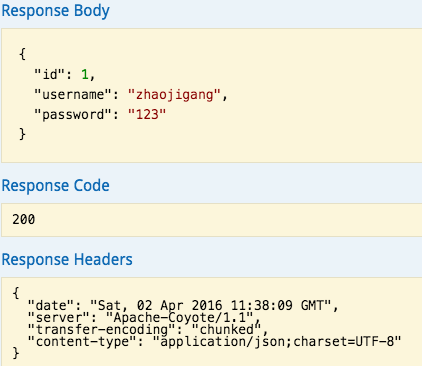