03.JDBC數據庫編程之處理DML語句
- void
- addBatch(String sql) Adds the given SQL command to the current list of commands for this Statement object.
- void
- clearBatch() Empties this Statement object's current list of SQL commands.
- void
- close() Releases this Statement object's database and JDBC resources immediately instead of waiting for this to happen when it is automatically closed.
- boolean
- execute(String sql) Executes the given SQL statement, which may return multiple results.
- int[]
- executeBatch() Submits a batch of commands to the database for execution and if all commands execute successfully, returns an array of update counts.
- ResultSet
- executeQuery(String sql) Executes the given SQL statement, which returns a single ResultSet object.
- int
- executeUpdate(String sql) Executes the given SQL statement, which may be an INSERT, UPDATE, or DELETE statement or an SQL statement that returns nothing, such as an SQL DDL statement.
- int
- executeUpdate(String sql, String[] columnNames) Executes the given SQL statement and signals the driver that the auto-generated keys indicated in the given array should be made available for retrieval.
- boolean
- isClosed() Retrieves whether this Statement object has been closed.
- boolean
- isCloseOnCompletion() Returns a value indicating whether this Statement will be closed when all its dependent result sets are closed.
- void
- setMaxRows(int max) Sets the limit for the maximum number of rows that any ResultSet object generated by this Statementobject can contain to the given number.
- void
- setQueryTimeout(int seconds) Sets the number of seconds the driver will wait for a Statement object to execute to the given number of seconds.
一、DML與Statement接口
1.DML(Data manipulation language)
數據庫操作語句,用於添加、刪除、更新和查詢數據庫紀錄,並檢查數據庫的完整性。常用的語句關鍵字主要包括insert、delete、update等。
(1)添加/修改/刪除表數據
增加一行數據:(思想:往哪張表添加?給哪幾行添加值?分別是什麼值?)
insert into 表名 (列1,列2,...,列n) values (值1,值2...,值n);
修改某行的列數據:(思想:改哪張表?你需要改幾列的值?分別改為什麼值?在哪些行生效?)
update 表名 set 列1=新值1, 列2=新值2,where expr;
刪除行:(思想:你要刪除哪張表的數據?你要刪掉哪些行?)
delete from 表名 where expr;
查看表數據:
select * from [表名];
(2)修改字段及屬性
修改表(表中的字段類型、字段名、添加字段、刪除字段、修改表名)
①修改表中字段屬性(不能修改字段名)
alter table [表名] modify [字段名] [字段類型] [約束條件] [fisrt|after 列名];
②修改表中字段名及屬性
alter table [表名] change [源字段名] [修改後的字段名] [字段類型] [約束條件] [fisrt|after 列名];
③增加表字段
alter table [表名] add [字段名] [字段類型] [約束條件] [first|after 列名];
④刪除表字段
alter table [表名] drop [字段名];
注意:[first|after 列名],用於修改字段的排序,其中,after將新增的字段添加在某一字段後;first表示將新建的字段放在該表第一列。
修改表名
命令:alter table [表名] rename to [新表名];
2.Statement接口
用於執行靜態的SQL語句並獲得返回結果的接口,通過Connection中的createStatement方法得到的
即,Statement stmt=conn.createStatement())
參考:http://docs.oracle.com/javase/8/docs/api/index.html
二、JDBC數據庫應用源碼實戰 1.源碼實現 任務:向連接的數據庫中靜態插入一條數據('dongguo',18,97).
import java.sql.*;
/*MySQL數據庫編程
* 實例(2):JDBC處理DML語句*/
public class JDBC_DML {
public static void main(String[] args) {
//0.數據庫URL、數據庫賬戶名稱與密碼
String url = "jdbc:mysql://localhost/jdbc_test_db";
String DBusername="root";
String DBpassword="896013";
//1.加載數據庫驅動程序到Java虛擬機
try{
Class.forName("com.mysql.jdbc.Driver"); //Driver為MySQL驅動類
}catch(ClassNotFoundException e)
{
System.out.println("找不到數據庫驅動程序類,加載驅動失敗!");
e.printStackTrace(); //將異常保存到log日志中
}
//2.創建Connection對象conn,表示連接到MySQL數據庫
Connection conn=null;
Statement stmt=null;
int i;
try{
conn=DriverManager.getConnection(url, DBusername, DBpassword);
//3.獲取能夠實現執行SQL語句的Statement對象
stmt=conn.createStatement();
//4.執行SQL語句,根據返回的int類型數據判斷是否插入成功
i=stmt.executeUpdate("insert into test (name,age,score) values ('dongguo',18,97)");
if(i!=1)
{
System.out.println("更新數據庫失敗!");
}
}catch(SQLException se)
{
System.out.println("連接數據庫失敗");
se.printStackTrace();
}
//6.關閉所有使用的JDBC對象,釋放JDBC資源
if(stmt!=null) //關閉聲明
{
try{
stmt.close();
}catch(SQLException e){
e.printStackTrace();
}
}
if(conn!=null) //關閉數據庫連接
{
try{
conn.close();
}catch(SQLException e){
e.printStackTrace();
}
}
}
}
運行結果 (1)插入一條記錄 stmt.executeUpdate("insert into test (name,age,score) values ('dongguo',18,97)");
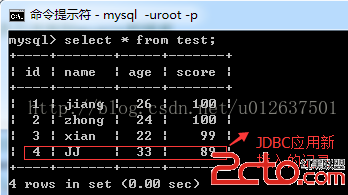
(2)刪除一條記錄 stmt.executeUpdate("delete from test where name='JJ'");
2.JDBC應用向數據庫動態插入數據 源碼:通過命令行的參數作為插入的數據源,即動態更新數據庫數據
import java.sql.*;
/
*MySQL數據庫編程
* 實例(3):JDBC處理DML語句.通過命令行輸入需要插入到數據庫中的數據*/
public class JDBC_DML2 {
public static void main(String[] args) {
if(args.length!=3) //輸入不正確,非正常退出
{
System.out.println( "Parament Error,Please Input Again!");
System.exit(-1);
}
String nameParam=args[0]; //獲取命令行第一個參數
int ageParam=Integer.parseInt(args[1]); //獲取命令行第二個參數,並轉換為整型
int scoreParam=Integer.parseInt(args[2]);//獲取命令行第三個參數,並轉換為整型
//0.連接數據庫相關參數
String url="jdbc:mysql://localhost:3306/jdbc_test_db"; //數據庫URL(資源定位唯一標識符)
String DBusername="root"; //數據庫用戶名
String DBpasswd="111111"; //數據庫密碼
//1.加載數據庫驅動,將Driver注冊到DriverManager中
try{
Class.forName("com.mysql.jdbc.Driver");
}catch(ClassNotFoundException e){
e.printStackTrace();
}
//2.通過數據庫URL連接到數據庫
Connection conn=null;
Statement stmt=null;
try{
conn=DriverManager.getConnection(url, DBusername, DBpasswd);
//3.獲取Statement對象
stmt=conn.createStatement();
//4.獲取命令參數,並調用Statement對象的executeUpdate更新數據庫
String sql="insert into test(name,age,score) values('"+nameParam+"',"+ageParam+","+scoreParam+")";
System.out.println(sql);//調試
stmt.executeUpdate(sql);
}catch(SQLException e){
e.printStackTrace();
}
//5.釋放JDBC資源
if(stmt!=null) //關閉聲明
{
try{
stmt.close();
}catch(SQLException e){
e.printStackTrace();
}
}
if(conn!=null) //關閉連接
{
try{
conn.close();
}catch(SQLException e){
e.printStackTrace();
}
}
}
}
(1)設置命令行參數 右擊工程->Run as->Open Run Dialog->Main Class選擇"JDBC_DML2"
(2)運行結果
(3)遇到的錯誤與調試 輸入參數運行Java應用後,出現SQLException異常。我們可以通過打印輸入的插入sql語句,發現程序沒有獲取到命令行傳入的參數,另外,vaules拼寫錯誤,找到原因。 修改:sql="insert into test(name,age,score) values('"+nameParam+"',"+ageParam+","+scoreParam+")";即可